Variable Assignment in Python
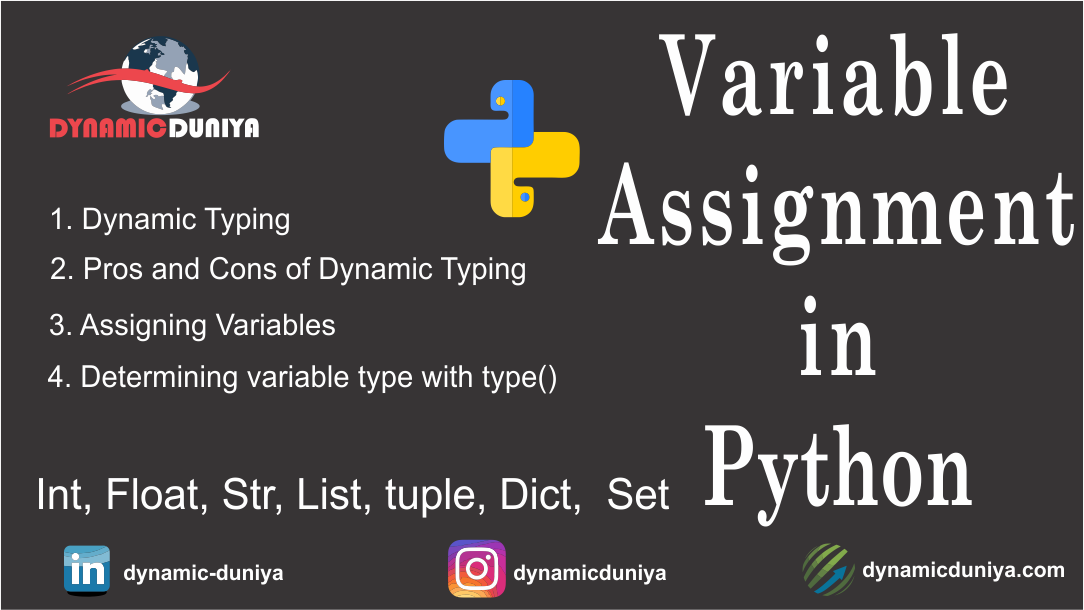
Python lets you create variables simply by assigning a value to the variable, without the need to declare the variable upfront. The value assigned to a variable determines the variable type. Different types may support some operations that others don't.
Rules for variable names
- names can not start with a number
- names can not contain spaces, use _ intead
names can not contain any of these symbols:
:'",<>/?|\!@#%^&*~-+
it's considered best practice (PEP8) that names are lowercase with underscores
- avoid using Python built-in keywords like
list
andstr
- avoid using the single characters
l
(lowercase letter el),O
(uppercase letter oh) andI
(uppercase letter eye) as they can be confused with1
and0
Dynamic Typing
Python uses dynamic typing, meaning you can reassign variables to different data types. This makes Python very flexible in assigning data types; it differs from other languages that are statically typed.
my_dogs = 2
my_dogs
my_dogs = ['Sammy', 'Frankie']
my_dogs
Pros and Cons of Dynamic Typing
Pros of Dynamic Typing
- very easy to work with
- faster development time
Cons of Dynamic Typing
- may result in unexpected bugs!
- you need to be aware of type()
Assigning Variables
The variable assignment follows name = object, where a single equals sign = is an assignment operator
a = 5
a
Here we assigned the integer object 5 to the variable name a. Let's assign a to something else:
a = 10
a
You can now use a in place of the number 10:
a + a
Reassigning Variables
Python lets you reassign variables with a reference to the same object.
a = a + 10
print(a)
There's actually a shortcut for this. Python lets you add, subtract, multiply and divide numbers with reassignment using +=, -=, *=, and /=.
a += 10
print(a)
#############
a *= 2
print(a)
Determining variable type with type()
You can check what type of object is assigned to a variable using Python's built-in type() function. Common data types include:
- int (for integer)
- float
- str (for string)
- list
- tuple
- dict (for dictionary)
- set
- bool (for Boolean True/False)
a=5
type(a)
a = (1,2)
type(a)
Simple Exercise
This shows how variables make calculations more readable and easier to follow.
my_income = 100
tax_rate = 0.1
my_taxes = my_income * tax_rate
print(my_taxes)
You should now understand the basics of variable assignment and reassignment in Python.
Random Blogs
- String Operations in Python
- Extract RGB Color From a Image Using CV2
- AI in Marketing & Advertising: The Future of AI-Driven Strategies
- Convert RBG Image to Gray Scale Image Using CV2
- Mastering SQL in 2025: A Complete Roadmap for Beginners
- The Ultimate Guide to Machine Learning (ML) for Beginners
- How to Start Your Career as a DevOps Engineer
- Types of Numbers in Python
- Role of Digital Marketing Services to Uplift Online business of Company and Beat Its Competitors
- Google’s Core Update in May 2020: What You Need to Know
Prepare for Interview
Datasets for Machine Learning
- Ozone Level Detection Dataset
- Bank Transaction Fraud Detection
- YouTube Trending Video Dataset (updated daily)
- Covid-19 Case Surveillance Public Use Dataset
- US Election 2020
- Forest Fires Dataset
- Mobile Robots Dataset
- Safety Helmet Detection
- All Space Missions from 1957
- OSIC Pulmonary Fibrosis Progression Dataset
- Wine Quality Dataset
- Google Audio Dataset
- Iris flower dataset
- Artificial Characters Dataset
- Bitcoin Heist Ransomware Address Dataset